Mastering Dependencies with Poetry: An Expert's Guide
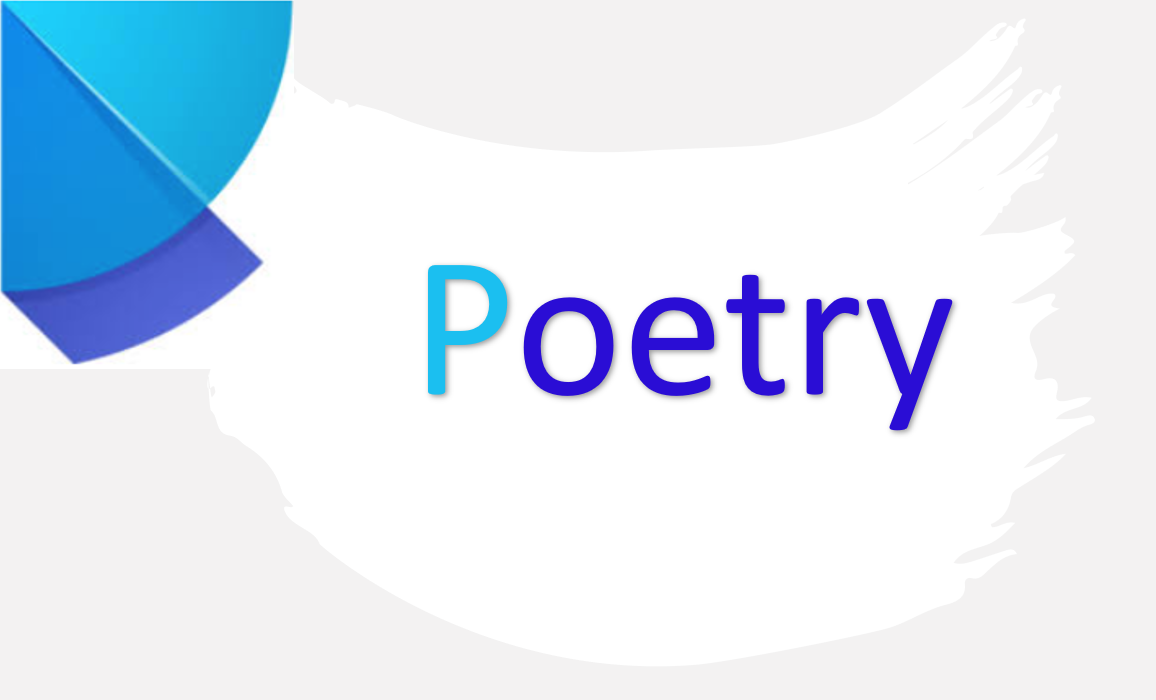
Introduction
Managing dependencies in a Python project can be a tedious task, especially as the project grows and the number of dependencies increases. This is where Poetry comes into play, a flexible and powerful dependency management tool that simplifies package management and dependency resolution. In this article, we’ll delve into some advanced features and practices of using Poetry in your Python projects.
Getting Started
Installation
Poetry can be easily installed using pip:
pip install --user poetry
Initializing a Project
Initialize a new project with Poetry:
poetry new my_project
cd my_project
Dependency Management
####Adding Dependencies Add a new dependency to your project:
poetry add numpy
Specifying Dependency Versions
Specify versions or version ranges for your dependencies:
[tool.poetry.dependencies]
python = "^3.8"
numpy = "^1.19"
Updating Dependencies
Update a specific dependency or all dependencies:
poetry update numpy
poetry update
Virtual Environments
Poetry creates a virtual environment for your project, ensuring dependencies are isolated.
Activating the Virtual Environment
poetry shell
Deactivating the Virtual Environment
exit
Packaging and Publishing
Building Your Package
Build your package with:
poetry build
Publishing Your Package
Publish your package to PyPi:
poetry publish --build
Advanced Usage
Custom Repository Sources
Add custom repository sources for dependency resolution:
[[tool.poetry.source]]
name = "private-repo"
url = "https://private-repo.example.com/simple/"
Dependency Groups
Create groups for optional dependencies:
[tool.poetry.group.dev.dependencies]
pytest = "^6.0"
Scripting with Poetry
Create custom scripts in your pyproject.toml:
[tool.poetry.scripts]
test = "pytest"
Run your custom script with:
poetry run test
Conclusion
Poetry provides an intuitive and robust way to manage dependencies, package, and publish your Python projects. Its ability to handle complex dependencies, provide isolated environments, and simplify package publishing makes it an indispensable tool for Python developers aiming to maintain clean and manageable projects.