Sailing the Clouds: Docker, GKE, and Terraform
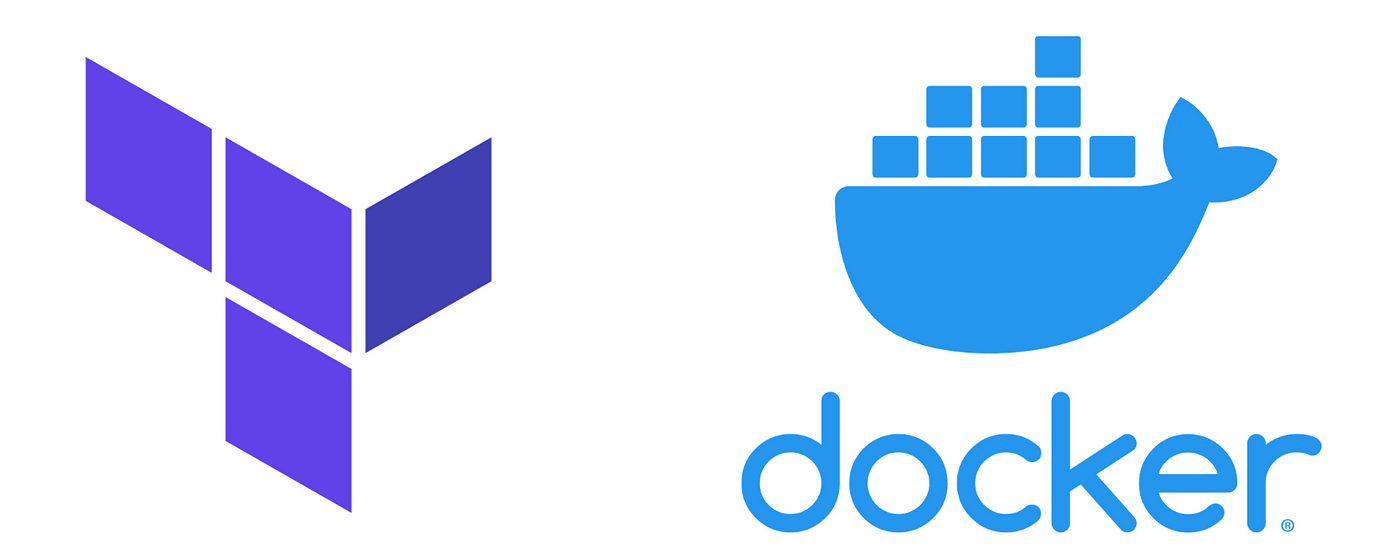
Introduction
The cloud-native ecosystem is bustling with tools that facilitate container orchestration, infrastructure as code, and seamless deployment. Among these tools, Docker, Google Kubernetes Engine (GKE), and Terraform stand out for their robustness and ease of use. This article unfolds the synergy between these tools and demonstrates how they can be leveraged to sail smoothly through the cloud-native waters.
Docker: Containerization at its Best
Docker is a platform that enables developers to create, deploy, and run applications in containers. Containers allow a developer to package up an application with all parts it needs, such as libraries and other dependencies, and ship it all out as one package.
Example: Dockerizing a Simple Application
# Create a Dockerfile
echo '
FROM python:3.8-slim-buster
WORKDIR /app
COPY requirements.txt requirements.txt
RUN pip install --no-cache-dir -r requirements.txt
COPY . .
CMD ["python", "app.py"]
' > Dockerfile
# Build the Docker image
docker build -t my-app .
GKE: Orchestrate Containers with Kubernetes
Google Kubernetes Engine (GKE) provides a managed environment for deploying, managing, and scaling your containerized applications using Google infrastructure. It leverages Kubernetes, the open-source container orchestration system.
Example: Deploying to GKE
# Create a Kubernetes deployment configuration
echo '
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-app-deployment
spec:
replicas: 3
selector:
matchLabels:
app: my-app
template:
metadata:
labels:
app: my-app
spec:
containers:
- name: my-app
image: gcr.io/my-project/my-app:latest
' > deployment.yaml
# Deploy to GKE
kubectl apply -f deployment.yaml
Terraform: Infrastructure as Code
Terraform is an open-source infrastructure as code software tool that enables users to define and provision a datacenter infrastructure using a high-level configuration language.
Example: Provisioning GKE Cluster using Terraform
provider "google" {
credentials = file("<YOUR-GCP-JSON-KEY>")
project = "<YOUR-GCP-PROJECT>"
region = "us-central1"
}
resource "google_container_cluster" "primary" {
name = "my-gke-cluster"
location = "us-central1-a"
remove_default_node_pool = true
initial_node_count = 1
master_auth {
username = ""
password = ""
client_certificate_config {
issue_client_certificate = false
}
}
}
output "cluster_endpoint" {
value = google_container_cluster.primary.endpoint
}
Conclusion
Embracing Docker, GKE, and Terraform can significantly streamline the deployment and management of cloud-native applications. By containerizing applications with Docker, orchestrating them with GKE, and provisioning infrastructure with Terraform, developers and operations teams can ensure consistency, scalability, and reliability across the development lifecycle.